diff --git a/docs/en/guides/heatmaps.md b/docs/en/guides/heatmaps.md
index 360f72ef..e11ee4d8 100644
--- a/docs/en/guides/heatmaps.md
+++ b/docs/en/guides/heatmaps.md
@@ -42,17 +42,43 @@ A heatmap generated with [Ultralytics YOLOv8](https://github.com/ultralytics/ult
# Heatmap Init
heatmap_obj = heatmap.Heatmap()
heatmap_obj.set_args(colormap=cv2.COLORMAP_CIVIDIS,
- imw=cap.get(4), # should same as im0 width
- imh=cap.get(3), # should same as im0 height
- view_img=True)
+ imw=cap.get(4), # should same as cap width
+ imh=cap.get(3), # should same as cap height
+ view_img=True)
while cap.isOpened():
success, im0 = cap.read()
if not success:
- exit(0)
+ print("Video frame is empty or video processing has been successfully completed.")
+ break
+
results = model.track(im0, persist=True)
im0 = heatmap_obj.generate_heatmap(im0, tracks=results)
+ cv2.destroyAllWindows()
+ ```
+
+ === "Heatmap with im0"
+ ```python
+ from ultralytics import YOLO
+ from ultralytics.solutions import heatmap
+ import cv2
+
+ model = YOLO("yolov8s.pt") # YOLOv8 custom/pretrained model
+
+ im0 = cv2.imread("path/to/image.png") # path to image file
+
+ # Heatmap Init
+ heatmap_obj = heatmap.Heatmap()
+ heatmap_obj.set_args(colormap=cv2.COLORMAP_JET,
+ imw=im0.shape[0], # should same as im0 width
+ imh=im0.shape[1], # should same as im0 height
+ view_img=True)
+
+
+ results = model.track(im0, persist=True)
+ im0 = heatmap_obj.generate_heatmap(im0, tracks=results)
+ cv2.imwrite("ultralytics_output.png", im0)
```
=== "Heatmap with Specific Classes"
@@ -70,17 +96,19 @@ A heatmap generated with [Ultralytics YOLOv8](https://github.com/ultralytics/ult
# Heatmap init
heatmap_obj = heatmap.Heatmap()
heatmap_obj.set_args(colormap=cv2.COLORMAP_CIVIDIS,
- imw=cap.get(4), # should same as im0 width
- imh=cap.get(3), # should same as im0 height
- view_img=True)
+ imw=cap.get(4), # should same as cap width
+ imh=cap.get(3), # should same as cap height
+ view_img=True)
while cap.isOpened():
success, im0 = cap.read()
if not success:
- exit(0)
+ print("Video frame is empty or video processing has been successfully completed.")
+ break
results = model.track(im0, persist=True, classes=classes_for_heatmap)
im0 = heatmap_obj.generate_heatmap(im0, tracks=results)
+ cv2.destroyAllWindows()
```
=== "Heatmap with Save Output"
@@ -102,18 +130,21 @@ A heatmap generated with [Ultralytics YOLOv8](https://github.com/ultralytics/ult
# Heatmap init
heatmap_obj = heatmap.Heatmap()
heatmap_obj.set_args(colormap=cv2.COLORMAP_CIVIDIS,
- imw=cap.get(4), # should same as im0 width
- imh=cap.get(3), # should same as im0 height
+ imw=cap.get(4), # should same as cap width
+ imh=cap.get(3), # should same as cap height
view_img=True)
while cap.isOpened():
success, im0 = cap.read()
if not success:
- exit(0)
- results = model.track(im0, persist=True, classes=classes_for_heatmap)
+ print("Video frame is empty or video processing has been successfully completed.")
+ break
+ results = model.track(im0, persist=True)
im0 = heatmap_obj.generate_heatmap(im0, tracks=results)
video_writer.write(im0)
+ video_writer.release()
+ cv2.destroyAllWindows()
```
=== "Heatmap with Object Counting"
@@ -133,17 +164,21 @@ A heatmap generated with [Ultralytics YOLOv8](https://github.com/ultralytics/ult
# Heatmap Init
heatmap_obj = heatmap.Heatmap()
heatmap_obj.set_args(colormap=cv2.COLORMAP_JET,
- imw=cap.get(4), # should same as im0 width
- imh=cap.get(3), # should same as im0 height
- view_img=True,
- count_reg_pts=count_reg_pts)
+ imw=cap.get(4), # should same as cap width
+ imh=cap.get(3), # should same as cap height
+ view_img=True,
+ count_reg_pts=count_reg_pts)
while cap.isOpened():
success, im0 = cap.read()
if not success:
- exit(0)
+ print("Video frame is empty or video processing has been successfully completed.")
+ break
results = model.track(im0, persist=True)
im0 = heatmap_obj.generate_heatmap(im0, tracks=results)
+
+ cv2.destroyAllWindows()
+
```
### Arguments `set_args`
diff --git a/docs/en/guides/index.md b/docs/en/guides/index.md
index e4ea7a86..e4efcea3 100644
--- a/docs/en/guides/index.md
+++ b/docs/en/guides/index.md
@@ -35,6 +35,8 @@ Here's a compilation of in-depth guides to help you master different aspects of
* [Objects Counting in Regions](region-counting.md) 🚀 NEW: Explore counting objects in specific regions with Ultralytics YOLOv8 for precise and efficient object detection in varied areas.
* [Security Alarm System](security-alarm-system.md) 🚀 NEW: Discover the process of creating a security alarm system with Ultralytics YOLOv8. This system triggers alerts upon detecting new objects in the frame. Subsequently, you can customize the code to align with your specific use case.
* [Heatmaps](heatmaps.md) 🚀 NEW: Elevate your understanding of data with our Detection Heatmaps! These intuitive visual tools use vibrant color gradients to vividly illustrate the intensity of data values across a matrix. Essential in computer vision, heatmaps are skillfully designed to highlight areas of interest, providing an immediate, impactful way to interpret spatial information.
+* [Instance Segmentation with Object Tracking](instance-segmentation-and-tracking.md) 🚀 NEW: Explore our feature on Object Segmentation in Bounding Boxes Shape, providing a visual representation of precise object boundaries for enhanced understanding and analysis.
+* [VisionEye View Objects Mapping](vision-eye.md) 🚀 NEW: This feature aim computers to discern and focus on specific objects, much like the way the human eye observes details from a particular viewpoint.
## Contribute to Our Guides
diff --git a/docs/en/guides/instance-segmentation-and-tracking.md b/docs/en/guides/instance-segmentation-and-tracking.md
new file mode 100644
index 00000000..f200cdbe
--- /dev/null
+++ b/docs/en/guides/instance-segmentation-and-tracking.md
@@ -0,0 +1,127 @@
+---
+comments: true
+description: Instance Segmentation with Object Tracking using Ultralytics YOLOv8
+keywords: Ultralytics, YOLOv8, Instance Segmentation, Object Detection, Object Tracking, Segbbox, Computer Vision, Notebook, IPython Kernel, CLI, Python SDK
+---
+
+# Instance Segmentation and Tracking using Ultralytics YOLOv8 🚀
+
+## What is Instance Segmentation?
+
+[Ultralytics YOLOv8](https://github.com/ultralytics/ultralytics/) Instance segmentation involves identifying and outlining individual objects in an image, providing a detailed understanding of spatial distribution. Unlike semantic segmentation, it uniquely labels and precisely delineates each object, crucial for tasks like object detection and medical imaging.
+Two Types of instance segmentation by Ultralytics YOLOv8.
+
+- **Instance Segmentation with Class Objects:** Each class object is assigned a unique color for clear visual separation.
+
+- **Instance Segmentation with Object Tracks:** Every track is represented by a distinct color, facilitating easy identification and tracking.
+
+## Samples
+
+| Instance Segmentation | Instance Segmentation + Object Tracking |
+|:---------------------------------------------------------------------------------------------------------------------------------------:|:------------------------------------------------------------------------------------------------------------------------------------------------------------:|
+| 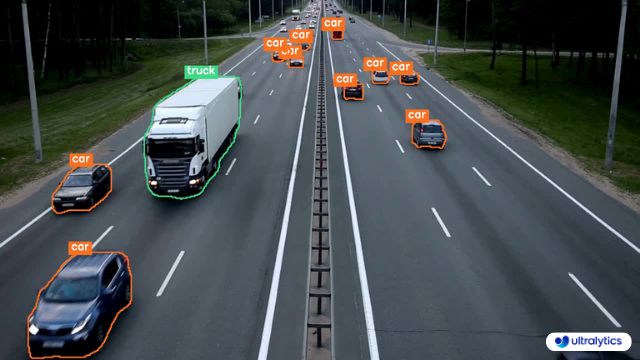 | 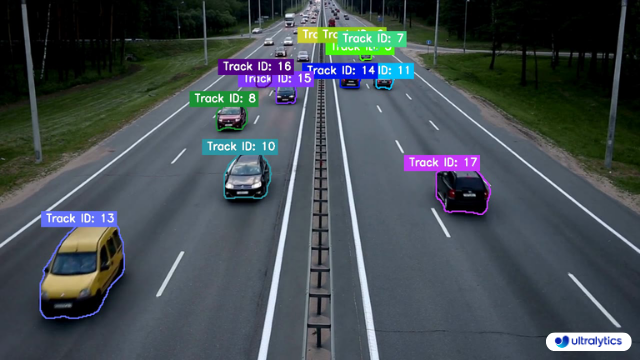 |
+| Ultralytics Instance Segmentation 😍 | Ultralytics Instance Segmentation with Object Tracking 🔥 |
+
+
+!!! Example "Instance Segmentation and Tracking"
+
+ === "Instance Segmentation"
+ ```python
+ import cv2
+ from ultralytics import YOLO
+ from ultralytics.utils.plotting import Annotator, colors
+
+ model = YOLO("yolov8n-seg.pt")
+ names = model.model.names
+ cap = cv2.VideoCapture("path/to/video/file.mp4")
+
+ out = cv2.VideoWriter('instance-segmentation.avi',
+ cv2.VideoWriter_fourcc(*'MJPG'),
+ 30, (int(cap.get(3)), int(cap.get(4))))
+
+ while True:
+ ret, im0 = cap.read()
+ if not ret:
+ print("Video frame is empty or video processing has been successfully completed.")
+ break
+
+ results = model.predict(im0)
+ clss = results[0].boxes.cls.cpu().tolist()
+ masks = results[0].masks.xy
+
+ annotator = Annotator(im0, line_width=2)
+
+ for mask, cls in zip(masks, clss):
+ annotator.seg_bbox(mask=mask,
+ mask_color=colors(int(cls), True),
+ det_label=names[int(cls)])
+
+ out.write(im0)
+ cv2.imshow("instance-segmentation", im0)
+
+ if cv2.waitKey(1) & 0xFF == ord('q'):
+ break
+
+ out.release()
+ cap.release()
+ cv2.destroyAllWindows()
+
+ ```
+
+ === "Instance Segmentation with Object Tracking"
+ ```python
+ import cv2
+ from ultralytics import YOLO
+ from ultralytics.utils.plotting import Annotator, colors
+
+ from collections import defaultdict
+
+ track_history = defaultdict(lambda: [])
+
+ model = YOLO("yolov8n-seg.pt")
+ cap = cv2.VideoCapture("path/to/video/file.mp4")
+
+ out = cv2.VideoWriter('instance-segmentation-object-tracking.avi',
+ cv2.VideoWriter_fourcc(*'MJPG'),
+ 30, (int(cap.get(3)), int(cap.get(4))))
+
+ while True:
+ ret, im0 = cap.read()
+ if not ret:
+ print("Video frame is empty or video processing has been successfully completed.")
+ break
+
+ results = model.track(im0, persist=True)
+ masks = results[0].masks.xy
+ track_ids = results[0].boxes.id.int().cpu().tolist()
+
+ annotator = Annotator(im0, line_width=2)
+
+ for mask, track_id in zip(masks, track_ids):
+ annotator.seg_bbox(mask=mask,
+ mask_color=colors(track_id, True),
+ track_label=str(track_id))
+
+ out.write(im0)
+ cv2.imshow("instance-segmentation-object-tracking", im0)
+
+ if cv2.waitKey(1) & 0xFF == ord('q'):
+ break
+
+ out.release()
+ cap.release()
+ cv2.destroyAllWindows()
+ ```
+
+### `seg_bbox` Arguments
+
+| Name | Type | Default | Description |
+|---------------|---------|-----------------|----------------------------------------|
+| `mask` | `array` | `None` | Segmentation mask coordinates |
+| `mask_color` | `tuple` | `(255, 0, 255)` | Mask color for every segmented box |
+| `det_label` | `str` | `None` | Label for segmented object |
+| `track_label` | `str` | `None` | Label for segmented and tracked object |
+
+## Note
+
+For any inquiries, feel free to post your questions in the [Ultralytics Issue Section](https://github.com/ultralytics/ultralytics/issues/new/choose) or the discussion section mentioned below.
diff --git a/docs/en/guides/object-counting.md b/docs/en/guides/object-counting.md
index c3407014..520f7dd2 100644
--- a/docs/en/guides/object-counting.md
+++ b/docs/en/guides/object-counting.md
@@ -10,6 +10,17 @@ keywords: Ultralytics, YOLOv8, Object Detection, Object Counting, Object Trackin
Object counting with [Ultralytics YOLOv8](https://github.com/ultralytics/ultralytics/) involves accurate identification and counting of specific objects in videos and camera streams. YOLOv8 excels in real-time applications, providing efficient and precise object counting for various scenarios like crowd analysis and surveillance, thanks to its state-of-the-art algorithms and deep learning capabilities.
+